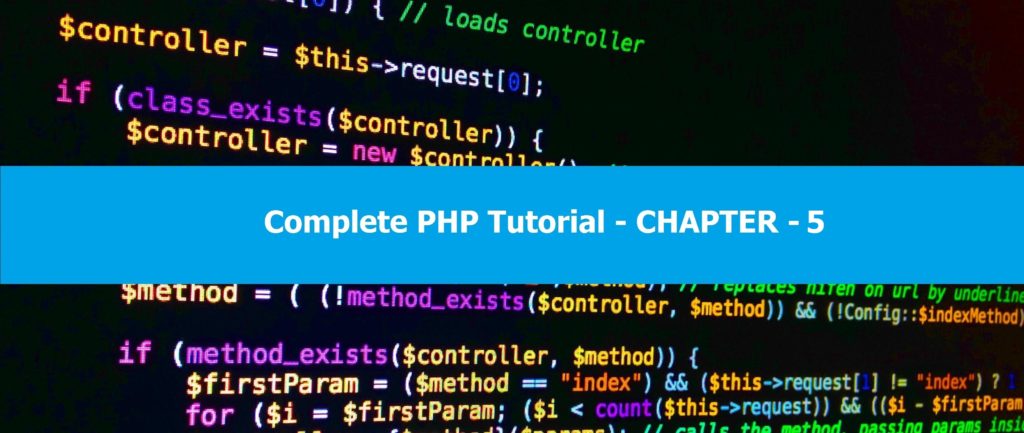
Here we’ll cover PHP Operators that are of various types like Arithmetic Operators, Assignment Operators, Logical Operators and Comparison Operators
Arithmetic Operators
Arithmetic operators work with numeric values to perform common arithmetic operations.
- Addition | $a + $b
- Subtraction | $a – $b
- Multiplication | $a * $b
- Division | $a / $b
- Modulus | $a % $b
- Exponentiation | $a ** $b
Most of these are self explanatory apart from modulus which some of you may not be familiar with. In short, it is the remainder of the division between the first and second operand. For example:
PHP Code:
<?php
$a = 14;
$b = 3;
echo $a % $b; // Outputs 2
?>
And if you are confused to what exponentiation is, it is simply what you get when you raise $a to the power $b. For example:
PHP Code:
<?php
$a = 13;
$b = 2;
echo $a ** $b; //Outputs 26
?>
You can also do increment’s and decrements. These can either be: post increment’s, pre increment’s, post decrements or previous decrements. To use a post increment simple follow the variable with ++ or — for a decrement.
PHP Code:
<?php
// Post increments.
$a++; // equivalent to $a = $a + 1
$a–; // equivalent to $a = $a – 1
?>
The difference with a post increment is that it returns the value before it changes the variable. Pre increments change the variable then change the value.
PHP Code:
<?php
$x = 5; $y = $x++; //$x = 6 and $y = 5
$x = 5; $y = $++x; //$x = 6 and $y = 6
?>
Assignment Operators
Assignment operators work with numeric values to write values to variables.
PHP Code:
<?php
$x = 35;
$x = $num1
?>
Assignment operators can also work with arithmetic operators.
PHP Code:
<?php
$x = 50;
$x += 10; // You can replace the + with any other arithmetic operator.
echo $x;
// Outputs 60
?>
Comparison Operators
Comparison operators allow you to compare two variables. They will return a Boolean answer of TRUE or FALSE. They are usually used inside of control structures which we will explain more about later. As for now, here is a list of all the comparison operators:
- Equal | ==
- Identical | ===
- Not Equal | !=
- Not Equal | <>
- Not Identical | !==
- Greater Than | >
- Greater Than or Equal To | >=
- Less Than | <
- Less Than or Equal To | <=
Logical Operators
Logical operators are used to combine conditional statements. Bellow is a list of all of them:
- And | and
- Or | or
- Xor | xor
- And | &&
- Or | ||
- Not | !
Continue to Next Chapter – Complete PHP Tutorial – Chapter 6
You may also like:- Mastering Windows Management with WMIC Commands – Top 20 Examples
- Edit and Compile Code with the Best 5 Code Editors
- 50+ Top DevSecOps Tools You Need To Know
- Learn How to Add Proxy and Multiple Accounts in MoreLogin
- Some Useful PowerShell Cmdlets
- Create Free SSL Certificate – ZEROSSL.COM [2020 Tutorial]
- Generate Self-Signed SSL Certificate with OPENSSL in Kali Linux
- RDP – CredSSP Encryption Oracle Remediation Solution 2020
- Scan Open Ports using Ss, Netstat, Lsof and Nmap
- Top 10 Dangerous Viruses of all times